Even though I have been working with C# for some time now, not mainly but consistently, I wasn’t necessarily aware (as I pointed out here) that there is in fact a difference when people refer to „appSettings“ and „applicationSettings“. This shall be a quick summary for fellow unknown „ignorants“.
Working on either larger backend applications that do not tend to store information locally (apart from the obligatory connectionString) or small helper applications that typically store a few settings, I considered there be only a single approach of using app.config for settings. When I started out, this was the approach I was given, and so far, happy with:
Head to your app.config and add a new „appSettings“ section as well as whatever you want to store:
<configuration> <appSettings> <add key="VeryImportantSetting" value="IsEnabled"/> </appSettings> </configuration>
Add a reference to System.Configuration
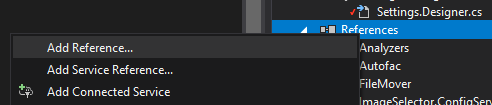

And then simply access the setting in code like this:
using System.Configuration; var setting = ConfigurationManager.AppSettings["VeryImportantSetting"]; if (setting) { DoSomething() }
Obviously, you would need to do some type checking but for the smaller needs this is minimalistic, quick and most important of all, effective.
What I did use back in the VB.NET times was a different approach which I successfully blocked from my memory. Within VisualStudio you can navigate to the project’s properties and will find a „Settings“ section:
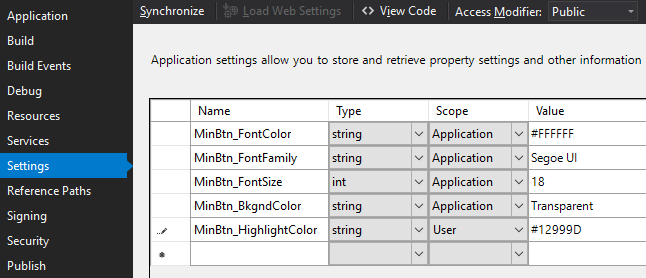
If you choose the Scope „user“ the setting will be stored in a user.config and stored somewhere in the AppData maze of the logged in user. For the sake of this comparision, let’s ignore that option. Choosing „Application“ as a scope will cause the setting you put in there to appear in your project’s app.config, like so:
<applicationSettings> <BestApplicationEver.Properties.Settings> <setting name="MinBtn_FontColor" serializeAs="String"> <value>#FFFFFF</value> </setting> <setting name="MinBtn_FontFamily" serializeAs="String"> <value>Segoe UI</value> </setting> <setting name="MinBtn_FontSize" serializeAs="String"> <value>18</value> </setting> <setting name="MinBtn_BkgndColor" serializeAs="String"> <value>Transparent</value> </setting> <setting name="MinBtn_HighlightColor" serializeAs="String"> <value>#12999D</value> </setting> </BestApplicationEver.Properties.Settings> </applicationSettings>
Accessing a setting would slightly different, like so:
int fSize = BestApplicationEver.Properties.Settings.MinBtn_FontSize;
While I definitely see the benefits of using a user scope for personliazed settings a desktop application, I find the latter way less attractive for standard settings or simple strings.
If you find yourself in a situation where you have to decide which approach to take, here is a incomplete list of pros and cons:
appSettings
+++
- very simple notation – almost no overhead. For the DevOps guys: very simple to add with NotePad on a server
- read/write access during application runtime
—
- no default values
- string data (some characters may not be supported; length is restricted)
- you have to do type checks
- no user scope
applicationSettings
+++
- support of default values
- application and user scope
- you can store complex objects in them (via serializeAs)
- as far as I am aware, no special character limitations
- you can directly reference them in XAML within your WPF application
—
- user settings stored somewhere in AppData of the user. Can be a pain to migrate or remove
- Application scoped settings are read only during runtime
Bottom line for me personally is that I prefer appSettings for my tools, with an exception of XAML related settings. I found that mixing both inside a single app.config works fine, so why not have the best of both worlds?
Hope that helps,
/chris